일부 연구 작업에서 연구원은 GPS 모듈을 사용하여 동물의 행동을 추적합니다. 1년 중 다른 시기에 다른 장소로 여행하는 방법을 추적할 수 있습니다.
이 예에서 우리는 이러한 종류의 데이터세트를 사용하여 새들이 다른 장소에서 어떻게 움직이는지 아이디어를 얻습니다. 이 데이터 세트에는 GPS 모듈의 위치 세부 정보가 저장되어 있습니다. 전체 데이터 세트는 CSV 형식입니다. 해당 파일에는 다른 필드가 있습니다. 첫 번째는 Bird Id, 다음은 date_time, 위도, 경도 및 속도입니다.
이 작업을 위해서는 Python 코드에서 사용할 수 있는 몇 가지 모듈이 필요합니다.
우리는 matplotlib, pandas 및 cartopy 모듈을 사용하고 있습니다. Anaconda에 설치하려면 다음 명령을 따르십시오. 필요할 때 다른 중요한 모듈을 설치합니다.
conda install -c conda-forge matplotlib conda install -c anaconda pandas conda install -c scitools/label/archive cartopy
처음에는 위도와 경도 값을 사용하여 위치를 플로팅합니다. 데이터세트에는 두 마리의 새가 있으므로 두 가지 색상을 사용하여 추적 위치를 시각화할 수 있습니다.
예시 코드
import pandas as pd from matplotlib import pyplot as plt df = pd.read_csv('bird_tracking.csv') cr = df.groupby('bird_id').groups cr_groups = df.groupby('bird_id') group_list = [] for group in cr: group_list.append(group) plt.figure(figsize=(7, 7)) #Create graph from dataset using the first group of cranes for group in group_list: x,y = cr_groups.get_group(group).longitude, cr_groups.get_group(group).latitude plt.plot(x,y, marker='o', markersize=2) plt.show()
출력
<중앙>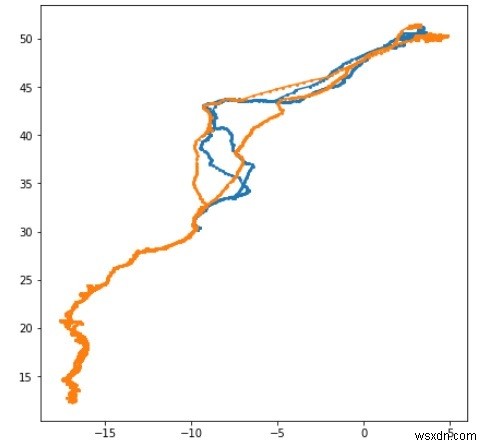
이제 이 추적 결과를 실제 지리 지도에 표시하여 새들이 사용하는 정확한 방법을 시각화할 수 있습니다.
예시 코드
import pandas as pd import cartopy.crs as ccrs import cartopy.feature as cfeature import matplotlib.pyplot as plt df = pd.read_csv("bird_tracking.csv") bird_id = pd.unique(birddata.bird_id) # Setup the projection to display the details into map projection = ccrs.Mercator() plt.figure(figsize=(7,7)) axes = plt.axes(projection=projection) axes.set_extent((-30.0, 25.0, 50.0, 10.0)) axes.add_feature(cfeature.LAND) axes.add_feature(cfeature.OCEAN) axes.add_feature(cfeature.COASTLINE) axes.add_feature(cfeature.BORDERS, linestyle=':') for id in bird_id: index = df['bird_id'] == id x = df.longitude[index] y = df.latitude[index] axes.plot(x,y,'.', transform=ccrs.Geodetic(), label=id) plt.legend(loc="lower left") plt.show()
출력
<중앙>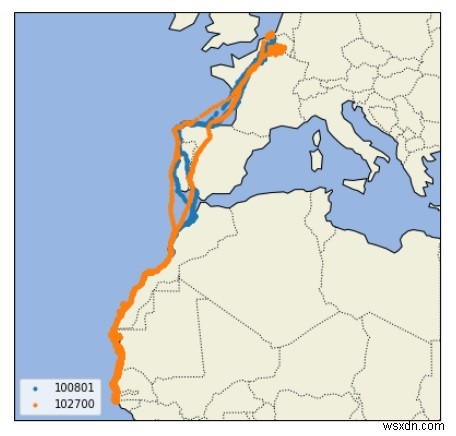